Introducing the LocalStack SDK for Python
LocalStack's new Python SDK allows users to interact directly with our internal developer endpoints using a Python-native interface. In this post, we’ll demonstrate how to use the SDK for seamless integration with LocalStack's features into your development and test pipelines.
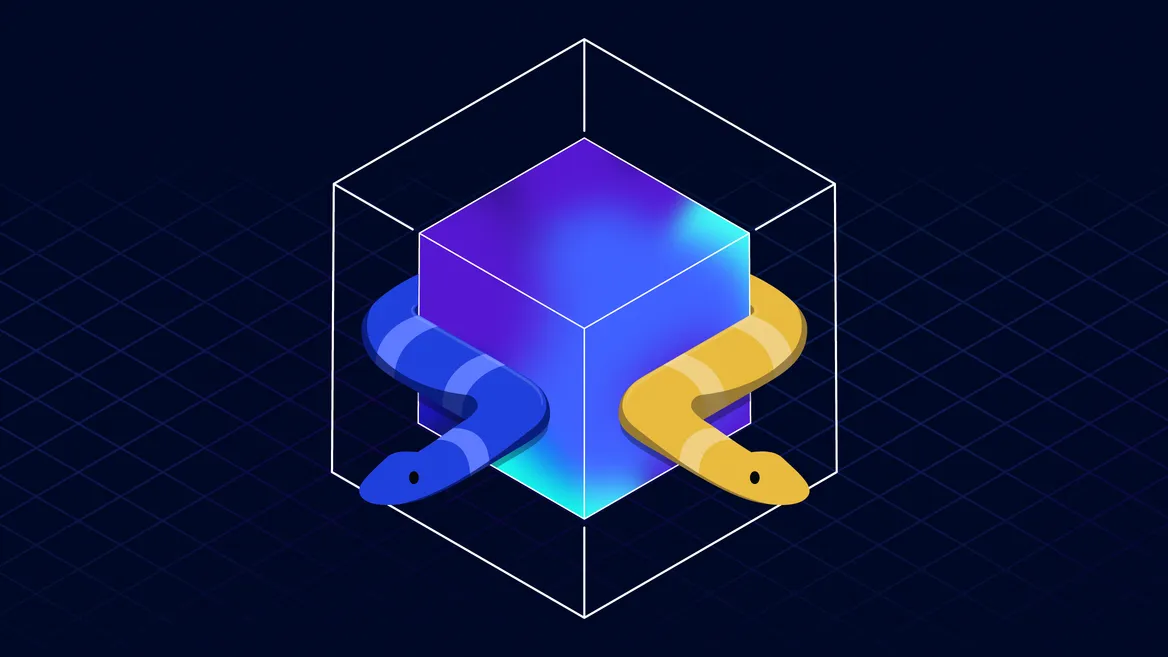
Introduction
One of the exiting announcements in LocalStack v3.8.0
was that we open-sourced our OpenAPI specification for LocalStack packages. This offers a RESTful experience for creating automations for LocalStack while reducing boilerplate code with the goal of enabling access to LocalStack features through simpler function calls via language SDKs.
This is why we’re excited to announce the release of the official LocalStack SDK for Python (preview). It provides a native, object-oriented interface to LocalStack’s internal endpoints. In this post, we’ll explain why and how to use the Python SDK in various development and testing scenarios with idiomatic Python programming paradigms.
Why use the LocalStack SDK for Python?
LocalStack exposes several developer endpoints for various tasks. These endpoints fall into two main categories:
- Internal endpoints (
_localstack/
path prefix): These provide access to LocalStack-specific features, such asPOST _localstack/pods/<name>
to create a new Cloud Pod on the platform. - AWS endpoints (
_aws/
path prefix): These enable you to access standard AWS features within LocalStack by exposing existing AWS APIs. For example,GET _aws/sqs/messages
offers a backdoor to view messages in SQS queues without side effects.
The LocalStack SDK for Python provides an easy way to access these operations in LocalStack’s API, providing methods for interacting with AWS-specific endpoints to control service behavior and proprietary features like Cloud Pods and the Chaos API.
The LocalStack SDK for Python is recommended if you’re looking to programatically interact with these endpoints to automate your workflow, eliminating the need to construct raw HTTP requests and parse responses, allowing for more intuitive and faster development.
Key Features
The LocalStack SDK for Python is designed to integrate seamlessly with the Python, LocalStack, and AWS cloud ecosystem. The key features include:
- Easy installation: Install the SDK from PyPI using
pip
. - CRUD support for Cloud Pods: Manage LocalStack’s state with ability to create, read, update, and delete Cloud Pods, as well as loading them via a PyTest fixture.
- Chaos API configuration management: Define disruption types for LocalStack’s Chaos API, to simulate outages on local infrastructure.
- State resets: Reset the LocalStack container state entirely using internal endpoints without manually restarting the container.
- AWS client operations: Interface with AWS SDK for Python (
boto3
) to perform certain operations like listing SQS queue messages or retrieving and deleting SES messages.
Getting started
Installing the LocalStack SDK for Python is as simple as running:
pip install localstack-sdk-python
The SDK organizes functionality into modules such as aws
, state
, pods
, and chaos
. For example, the aws
module lets developers initialize clients for various AWS services.
Developers can easily use the SDK by importing the required modules and initializing specific clients (e.g., AWSClient
, StateClient
, PodsClient
, ChaosClient
) to perform actions on local AWS services. For example, you can use the SDK to initialize the LocalStack AWS client, and then setup the S3 client using the LocalStack AWS client configuration:
import boto3import localstack.sdk.aws
client = localstack.sdk.aws.AWSClient()
s3_client = boto3.client( "s3", endpoint_url=client.configuration.host, region_name="us-east-1", aws_access_key_id="test", aws_secret_access_key="test",)
Once configured, you can create an S3 bucket and list all S3 buckets in the LocalStack container:
bucket_name = "test-bucket"s3_client.create_bucket(Bucket=bucket_name)print(f"Bucket '{bucket_name}' created.")
# List all S3 bucketsresponse = s3_client.list_buckets()buckets = response.get("Buckets", [])if buckets: print("Buckets:") for bucket in buckets: print(f" - {bucket['Name']}")else: print("No buckets found.")
If you’re using the LocalStack Pro image and want to access proprietary services or features, export your LOCALSTACK_AUTH_TOKEN
in your terminal before running the code.
Use Cases
Next, let’s explore some use cases for the LocalStack SDK for Python. Internally, we’ve been using the SDK in our projects and sample applications to simplify interactions with LocalStack-specific features, streamline developer workflows, and enable efficient, collaborative cloud application development.
Pre-seeding testing environments
One of the key use cases for LocalStack is its use in test environments, both locally and in CI pipelines. Automated testing often requires creating fixtures or resources to bootstrap the environment. Cloud Pods simplify this process by providing state snapshots of the LocalStack container, allowing users to selectively save and load resource state, which can be used to bootstrap a testing environment.
Using the LocalStack SDK for Python, the PodsClient
makes it easy to interact with the Cloud Pods API to list, save, load, and delete Cloud Pods. Here’s an example:
from localstack.sdk.pods import PodsClient
POD_NAME = "localstack-sdk-cloud-pod"client = PodsClient()
# List all podspods = client.list_pods()print("Pods:", pods)
# Save Cloud Podclient.save_pod(pod_name=POD_NAME)print(f"Pod '{POD_NAME}' saved.")
# Load Cloud Podclient.load_pod(pod_name=POD_NAME)print(f"Pod '{POD_NAME}' loaded.")
# Delete Cloud Podclient.delete_pod(pod_name=POD_NAME)print(f"Pod '{POD_NAME}' deleted.")
If you already have a defined Cloud Pod to seed data in your test environment, you can use the @cloudpods
decorator to load the specified Cloud Pod, run the test, and reset the LocalStack state afterward:
from localstack.sdk.testing import cloudpods
@cloudpods(name="seed-pod")def test_my_app(): # write your test logic here ...
This approach offers powerful capabilities for streamlining test setup, and we’ll explore it further in a follow-up blog post!
Running simulated infrastructure outages
LocalStack Chaos API enables you to simulate outages for any AWS region or service. It allows you to configure API failures by specifying the service, region, operation, HTTP error code, and error message. This feature lets you trigger local service outages to monitor system responses and test your application’s resiliency.
Using the LocalStack SDK for Python, the ChaosClient
simplifies interacting with the Chaos API. You can add fault rules for specific services, list applied rules, and delete rules to stop ongoing outages. Here’s an example to simulate a local outage for a DynamoDB table:
from localstack.generated import FaultRulefrom localstack.sdk.clients import ChaosClient
def initiate_dynamodb_outage() -> FaultRule: outage_rule = FaultRule(region="us-east-1", service="dynamodb") chaos_client = ChaosClient() rules = chaos_client.add_fault_rules(fault_rules=[outage_rule]) return rules
def check_outage_status(expected_status: FaultRule): chaos_client = ChaosClient() outage_status = chaos_client.get_fault_rules() assert outage_status == expected_status
def stop_dynamodb_outage(): chaos_client = ChaosClient() chaos_client.set_fault_rules(fault_rule=[]) check_outage_status([])
def test_dynamodb_outage(dynamodb_resource): outage_payload = initiate_dynamodb_outage() # write your test logic here
You can explore a complete test case scenario in one of our sample apps to better understand how to leverage the Chaos API with our SDK.
Verify delievered SES emails
LocalStack tracks all sent SES emails in memory for retrospection and provides a service endpoint to retrieve saved messages. You can use query parameters like ID
and email
to filter messages by ID or source email.
With the LocalStack SDK for Python, you can fetch these emails or clear them entirely from memory. Here’s an example:
import boto3import localstack.sdk.aws
client = localstack.sdk.aws.AWSClient()
# Initialize SES clientses_client = boto3.client( "ses", endpoint_url=client.configuration.host, region_name="us-east-1", aws_access_key_id="test", aws_secret_access_key="test",)
# Verify email addressemail = "user@example.com"ses_client.verify_email_address(EmailAddress=email)
# Send a raw emailraw_message_data = f"From: {email}\nTo: recipient@example.com\nSubject: test\n\nThis is the message body.\n\n"ses_client.send_raw_email(RawMessage={"Data": raw_message_data})
# Get and print SES message IDsmessages = client.get_ses_messages()for msg in messages: print("Message ID:", msg.id)
# Discard all SES messagesclient.discard_ses_messages()
Learn more
Check out these other resources to learn more about the LocalStack SDK for Python:
- Quickstart guide: To get started with our SDK, check out the official documentation, including examples.
- Repo on GitHub:
localstack-sdk-python
is open source on GitHub. Star the repo to follow along! - Check out our product changelog for all the information on the latest releases and how you can use them.
Conclusion
We’d love for you to give the LocalStack SDK for Python a shot and let us know what you think. Please keep in mind that it is currently in preview and may undergo fast and breaking changes. We believe that it will be a powerful addition to our developer tools, offering an object-oriented interface and a clean, intuitive way to access LocalStack’s advanced capabilities to enhance local cloud development and testing. Going ahead, our plan is to extend the SDK support and have maximum interoperatibility for popular Python testing frameworks like pytest
and TestContainers for Python.
Moving forward, all updates will be published to the SDK’s package on PyPI. If you have any feedback or suggestions, feel free to reach out (as an issue on the SDK repository or on our Slack community). Your input helps us improve and tailor the SDK to better meet your needs.